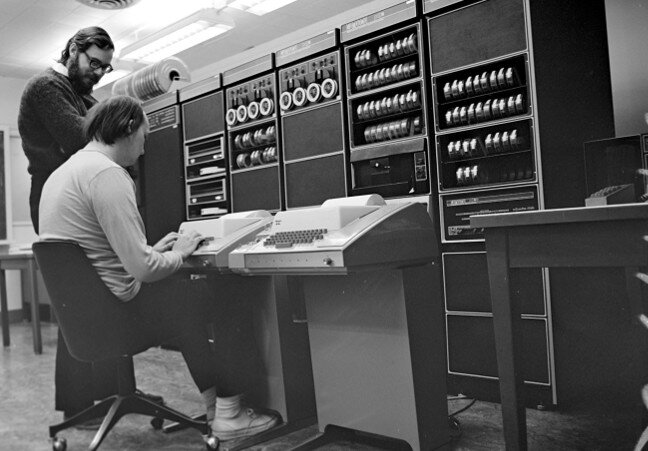
Brian Kernighan (left), who invented “Hello, World!” with the two creators of C programming: Ken Thompson (right) and Dennis Ritchie. Kernighan is originally from Toronto, Canada, and is known to coin the expression “What You See Is All You Get” (WYSIAYG) as it relates to the sensitivities of programming language.
What is a computer program?
People think of coding as some sort of wizardry magic where we use a computer and some program and it magically spits out a number. And honestly, that’s not too far off from the truth, the only difference is that it isn’t magic at all, it’s a set of instructions that someone else wrote so you don’t have to think about it.
What are computer Inputs?
A computer Input can be anything that is provided to a computer program for processing.
An Input could be from a person or even a machine.
When we’re talking about inputs, we mean any type of data that enters a computer program. For example, when you sign up for a platform like TikTok you enter your username and account details which the app uses later to display on your profile.
What are computer Outputs?
A computer Output is what the program displays or shares once it’s done processing any inputs
An Output can be intended for people, machines or even for another part of the same program.
Outputs can really be a variety of different things. In fact it’s not even necessary to have an input to create an output. All it means is the computer program generates something and is sharing it with some external source.
Coding your first Output in C — Hello World.
The historical explanation of Hello World is ensuring that a language is working correctly. Hello World is known as the most famous program which will tell the computer to display the two words “ Hello, World!”. Hit the run button to see the output in the console.
The code above is completely editable, so give it a shot by changing the “Hello World“ text in the editor and hit run to see your changed output.
At this point you’re probably wondering what each line in that code editor means, so let’s break it down.
#include
The first line of the program tells our program which utilities to include. Imagine someone made a piece of code and by using include you’re telling your program that you want to use that piece of code. A collection of code is called a Library. In this instance we’re telling our program to include the stdio.h library.
int main(void){ ~your code goes here~ }
Remember we talked about pieces of code? Well this is what a piece of code looks like, specifically a Function. A function is a set of steps that will be executed every-time that piece of code (or function) is called. All the list of steps are wrapped inside curly brackets so the function knows when to start and when to end. The unique thing about the main function is that whenever the program starts it will call this function, that’s why you don’t see this function be called anywhere. We’ll cover more on this and what int and void are in future tutorials.
printf(“Hello World“);
On the topic of Functions and Libraries, we have an example of a function that is from the library we imported at the top of the code. The print function gives your program the ability to output whatever text you put into its parenthesis. In this case, we give the function “Hello World“ as a parameter which it uses to output into the console.
Aside: It’s not about the code, it’s about the approach.
Understand the problem
New coders will often times get wrapped up in “how can I code this in C” or “am I naming my variables the right way”. Unfortunately this approach leads to overthinking details that are all solvable and overtime they will become like muscle memory.
When you’re starting off you need to make sure that you fully understand the problem and have the steps needed to solve before you even start coding. A great way to practice this type of approach is by writing the problem and solution out on a piece of paper. Create a list of steps between the problem and solution then use those same steps to translate into code.
Find a solution even if it’s not the most elegant, then work on improving it over time
It’s rarely the case that the first solution is the best solution, so don’t even bother coming up with the perfect solution on the first run. Create a basic solution in the beginning then iterate on it to make it more robust / efficient.
Account for uncommon scenarios
The honest truth is that what you’re expecting a user to do when using your program is probably not encompassing what could happen. You need to account for all the possible scenarios so that when someone else uses your program they don’t get some unexpected results. This may seem like a small point considering all we’ve done so far is write “Hello world“ into the console, but it’s a critical part to coding and you should always keep this in mind whenever you’re thinking of a solution.
Bad syntax can break your code!
As you can see after pressing run, the console tells us that there is an error. In this specific case it’s a Syntax error. Syntax is a fancy word that means the key characters that are used to code. For example, as we learned earlier every function needs to be wrapped in curly brackets. The important thing to note is that every language has its own syntax. A great way we can start learning a language is by looking at the syntax documentation for that language.
By looking carefully at the errors we can try to debug them. It says 4:25: error which means on line 4 and character 25 we have an error. Now you can get your desired result by adding a parentheses after “ to match it with 4:29 which is where the parentheses started.
Coding your first Input in C — Hello World!
Reading and writing comments like a PRO.
Comments can help you to explain the code that you write by adding some description on top of your code. Whatever you write as comments will not show in the results of your code.
By pre-fixing and line with two forward slashes we can tell the program to not execute those lines of code. In the example above you can see that we left a comment for every line of code that’s written in the function. What can you learn by reading the comments in the code?
Next steps.
Now that you’ve learned a few things about how to create inputs and outputs, we can move forward on our journey to becoming an expert thinker! The most important takeaway is understanding how you will approach problem solving and how this can vastly accelerate your coding skills.
You can now use what you just learned to go onto the next tutorial and learn about variables and integers to build more complex programs that take a variety of inputs.